제 5장 변수와 연산자 - 2
참고 : 개발툴 ( Dev C++)
● 상수란?: 변경이 불가능한 수 // 변수와는 구분됨
ex) 1, 2, 3...등등
● 산술연산자와 대입연산자
- 산술 연산자의 종류
ex) +, -, *, / , % 등이 있다 (더하기, 빼기, 곱하기, 나누기, 나머지 구하기)
===========================================
#include <stdio.h>
int main()
{
int num1 = 10; // 변수 선언동시에 10으로 초기화
int num2 = 3; // 변수 선언동시에 3으로 초기화
printf("두수의 더하기 : %d \n", num1 + num2);
printf("두수의 빼 기 : %d \n", num1 - num2);
printf("두수의 곱하기 : %d \n", num1 * num2);
printf("두수의 나누기 : %d \n", num1 / num2);
printf("두수의 나머지 : %d \n", num1 % num2);
system("PAUSE");
return 0;
}
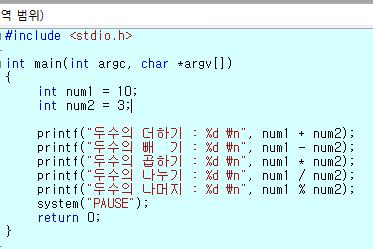
===========================================
● 기타 대입연산자
- 대입연산자와 산술연산자가 합해져서 다양한 형태의 대입연산자 정의
ex) +=, -=, *=, /=, %=
ex) a = a+a; == a+=a;
a = a-a; == a-=a;
a = a*b; == a*=b;
a = a/b; == a/=b;
==========================================
#include <stdio.h>
int main()
{
int val1 = 3;
int val2 = 4;
int val3 = 5;
val1 += 3; // val1 = val1 + 3;
val2 -= 4; // val2 = val2 + 4;
val3 *= 5; // vla3 = val3 + 5;
printf("rusult : %d %d %d \n", val1, val2, val3);
system("PAUSE");
return 0;
}
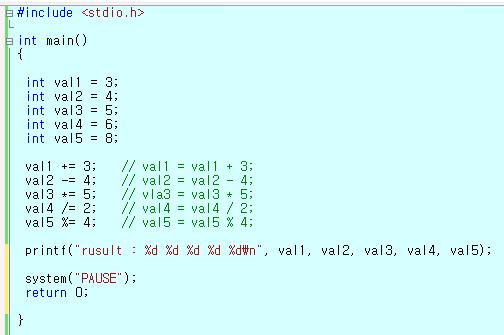
===========================================
● 다양한 연산자(수학적 지식??산수적 지식이란 같음)
부호 연산으로서 +, - 연산자
ex) -(+20) = -20
-(-20) = +20
===========================================
#include <stdio.h>
int main()
{
int number = 8;
int number2 = -10;
number1 = -number1;
printf("number1 = -number1 연산후 : %d \n", number1);
number2 = -number2;
printf("number2 = -number2 연산후 : %d \n", number2);
system("PAUSE");
return 0;
}
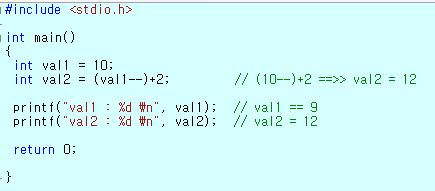
===========================================
※ 증감감소연산자
1. ++ : 증가 연산자(+1 증가)
2. -- : 감소 연산자(-1 감소)
ex) ++a : 1 먼저 증가 시킨후, 연산을 실행
a-- : 먼저 연산 후, 감소시킨다.
===========================================
#include <stdio.h>
int main()
{
int val1 = 10;
int val2 = (val1--)+2; // (10--)+2 ==>> val2 = 12
printf("val1 : %d \n", val1); // val1 == 9
printf("val2 : %d \n", val2); // val2 = 12
return 0;
}
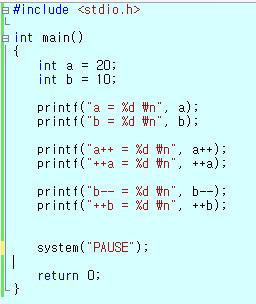
#include <stdio.h>
int main()
{
int a = 20;
int b = 10;
printf("a = %d \n", a); // a = 20
printf("b = %d \n", b); // b = 10
printf("a++ = %d \n", a++); // a를 먼저 %d로(%d = 20) 그리고 난후 1 증가
printf("++a = %d \n", ++a); // a를 1증가 (a = 22) 그리고 난후 대입
printf("b-- = %d \n", b--);
printf("++b = %d \n", ++b);
system("PAUSE");
return 0;
}
● 관계연산자(비교 연산자)
- 논리적 참일때 : true(1);
- 논리적 거짓일때 : false(0);
- 사실은 컴퓨터는 0이 아닌값은 모두 true 라고 본다.
ex) -1, -34, 45, 643 모두 true 다.
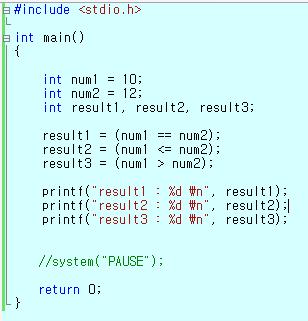
● 논리 연산자
- and, or, not을 표현하는 연산자
- true(1), false(0) 반환
1. && (and연산): 두 관계가 둘다 참일때 true 리턴
2. || (or연산) : 두 관계중 하나라도 참일때 true 리턴
3. !(not 연산) : 이 연산의 참이면 false 리턴, 거짓이면 true 리턴
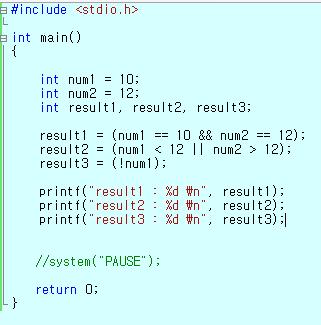
@ 연산자 우선순위로 실행되지만
- 우선은 우리가 알고 있는 수학적 연산순위와 크게 다를건 없다.
● 콤마 연산자
- 둘이상의 변수 동시 선언
- 둘 이상의 문장을 한줄에 선언 시
- 함수의 매개변수 전달 시
-연산자의 결합성
ex) 1+4*3/2-6
=> +, - 보다는 *, / 연산이 먼저 실행이 된다.
=> 1+(4*3)(/2)-6;
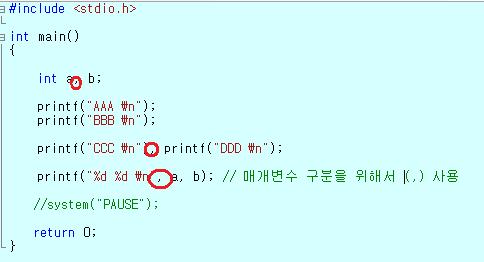
● 대입연산자
int a, b, c;
a = b = c = 10;
printf("%d %d %d \n", a, b, c);
'프로그래밍 > C' 카테고리의 다른 글
제 7장 비트단위 연산과 상수, 기본자료형 (0) | 2010.07.08 |
---|---|
제 6장 scanf함수와 데이타 표현방식의 이해 (0) | 2010.07.08 |
제 4장 변수와 연산자 (0) | 2010.07.08 |
제 3장 C언어의 기초 - printf함수 (0) | 2010.07.08 |
제 2장 C언어의 기초 (0) | 2010.07.08 |